The final step of building a machine learning (ML) model is deployment, which makes the model available to end-users or systems. Model deployment involves preparing the trained ML model for real-world use.
This blog will explain model deployment using a Flask API on Heroku. In Part-1, we will create the necessary files. In Part-2, we will upload these files to GitHub and run the app on Heroku.
What is Flask API?
Flask is a Python web framework that simplifies web application development. It is easy to use and provides features like integrated unit testing, a built-in development server, and debugging tools.
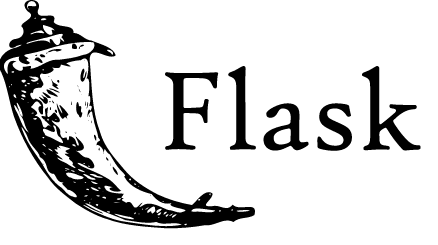
Necessary Files for Deployment
To prepare the model for deployment, create these files:
- model.py: Contains the code for training the ML model and making predictions.
- model.pkl: A serialized version of
model.py
created using thepickle
module. It allows the model to be reused in other Python scripts. - app.py: Handles user inputs via GUI or API calls, computes predictions, and returns results.
- request.py: Sends API requests defined in
app.py
and displays the results. - Template folder: Contains the HTML template for user inputs. Add a CSS file here for styling, if needed.
- requirements.txt: Lists the Python packages required to run the app.
- Procfile: Specifies the type of app and how Heroku should serve it.
Step 1: Training and Tuning the Model
Train your model and save it as model.py
. Below is an example using the RandomForestClassifier to predict tree types based on five features.
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
dataset = pd.read_csv('final.csv')
X = dataset.drop("Cover_Type", axis=1)
y = dataset["Cover_Type"]
classifier = RandomForestClassifier()
classifier.fit(X, y)
Save this model in model.py
to enable predictions.
Step 2: Pickling (Serialization)
Serialize the trained model using the pickle
module. Serialization saves the model for future use, while deserialization reloads it.
import pickle
# Serialize
pickle.dump(classifier, open('model.pkl', 'wb'))
# Deserialize
model = pickle.load(open('model.pkl', 'rb'))
print(model.predict([[2788, 2, 3555, 221, 2984]]))
Step 3: Creating app.py
Create app.py
to process inputs, make predictions, and return results. Below is an example:
from flask import Flask, request, render_template, jsonify
import pickle
import numpy as np
app = Flask(__name__)
model = pickle.load(open('model.pkl', 'rb'))
@app.route('/')
def home():
return render_template('index.html')
@app.route('/predict', methods=['POST'])
def predict():
int_features = [int(x) for x in request.form.values()]
final_features = [np.array(int_features)]
prediction = model.predict(final_features)
return render_template('index.html', prediction_text=f'Tree type is {prediction[0]}')
@app.route('/results', methods=['POST'])
def results():
data = request.get_json(force=True)
prediction = model.predict([np.array(list(data.values()))])
return jsonify(prediction[0])
if __name__ == '__main__':
app.run(debug=True)
Step 4: Creating request.py
The request.py
file sends API requests to app.py
and displays predictions.
import requests
url = 'http://localhost:5000/results'
data = {"Elevation": 2434, "Vertical_Distance_To_Hydrology": 80, "Horizontal_Distance_To_Roadways": 234, "Hillshade_Noon": 221, "Horizontal_Distance_To_Fire_Points": 469}
response = requests.post(url, json=data)
print(response.json())
Step 5: Creating requirements.txt
and Procfile
1. requirements.txt: Lists the necessary Python packages for the app in shell.
Flask==1.1.1
gunicorn==19.9.0
numpy>=1.9.2
scikit-learn>=0.18
pandas>=0.19
2. Procfile: Instructs Heroku on how to run the app.
Now we have completed creating the necessary files for model deployment. Uploading these files to GitHub and how to create an app from them will be covered in Model Deployment With Flask/Part-2.
Summary
- Model deployment makes ML models accessible to users.
- This blog focuses on deploying models using Flask and Heroku.
- In Part-1, we prepared the required files:
model.py
,model.pkl
,app.py
,request.py
,requirements.txt
, and Procfile. - Part-2 will cover uploading the files to GitHub and running the app on Heroku.